Advanced React JS Concepts: A Deep Dive
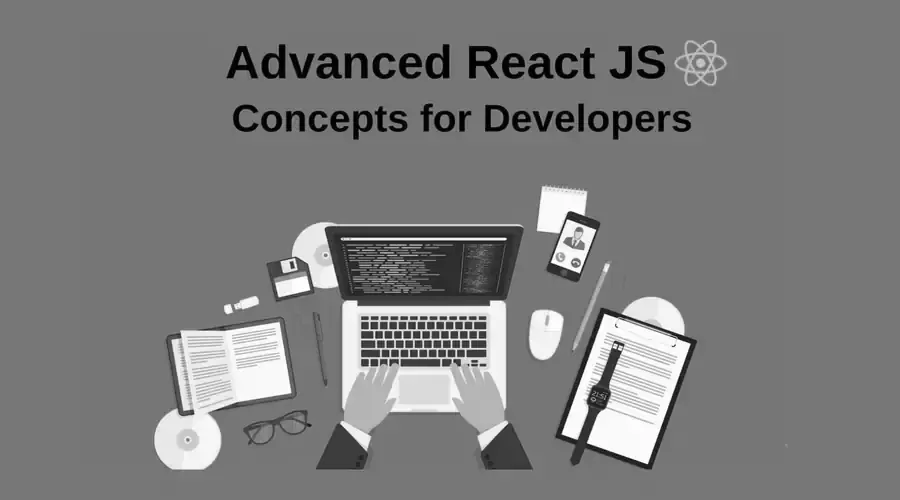
- Published on
- /4 mins read/βββ views
π Introduction
React JS has revolutionized web development by providing a flexible and efficient way to build interactive user interfaces. Whether you're a beginner or an experienced developer, understanding Reactβs core concepts and advanced techniques will help you build high-performing applications. In this guide, we cover everything from React basics to optimization strategies that ensure your application is scalable, maintainable, and SEO-friendly.
π― React JS Basics: Understanding the Fundamentals
π Components and JSX
React applications are built using components, which serve as reusable building blocks. These components encapsulate logic and UI elements, making development more modular and efficient.
πΉ JSX (JavaScript XML) is a syntax extension for JavaScript that makes writing UI components intuitive by using HTML-like code. JSX improves code readability and maintains a clean structure.
const Greeting = () => <h1>Hello, World!</h1>
π State and Props
React components manage data using state and props:
- State: Holds dynamic data within a component. When state updates, React automatically re-renders the component.
- Props: Short for "properties," props allow data to be passed from parent to child components.
const Welcome = (props) => <h1>Welcome, {props.name}!</h1>
β‘ Virtual DOM
React optimizes performance by using a virtual DOM, a lightweight copy of the real DOM. When changes occur, React compares the new virtual DOM with the previous one and updates only the modified parts, improving rendering efficiency.
π₯ Advanced React Concepts: Elevating Your Skills
π React Hooks
Introduced in React 16.8, Hooks enable functional components to manage state and lifecycle behavior without needing class components.
useState
: Manages local component state.useEffect
: Handles side effects like API calls and event listeners.
import { useState } from 'react'
const Counter = () => {
const [count, setCount] = useState(0)
return <button onClick={() => setCount(count + 1)}>Count: {count}</button>
}
π Context API: Managing Global State
The Context API eliminates "prop drilling" by providing a global state accessible by any component.
const UserContext = React.createContext()
const App = () => (
<UserContext.Provider value={{ name: 'John Doe' }}>
<Profile />
</UserContext.Provider>
)
π React Router: Client-Side Navigation
React Router enables single-page applications to handle multiple routes efficiently.
import { BrowserRouter, Route, Routes } from 'react-router-dom'
;<BrowserRouter>
<Routes>
<Route path="/home" element={<Home />} />
<Route path="/about" element={<About />} />
</Routes>
</BrowserRouter>
π Optimizing React Performance
useMemo
ποΈ Memoization with Memoization prevents expensive calculations from running on every render.
const expensiveCalculation = useMemo(() => computeHeavyLogic(data), [data])
React.lazy
π€ Lazy Loading with Load components only when needed, reducing initial bundle size.
const LazyComponent = React.lazy(() => import('./LazyComponent'))
π₯ Code Splitting for Faster Load Times
Code splitting dynamically loads JavaScript files, improving performance.
import('./module').then((Module) => Module.default())
π React Testing: Ensuring Code Quality
β Unit Testing with Jest
Jest allows writing automated test cases to verify individual component behavior.
test('renders welcome message', () => {
render(<Welcome name="React" />)
expect(screen.getByText(/Welcome, React!/i)).toBeInTheDocument()
})
π Integration Testing with React Testing Library
Simulate user interactions and test how components work together.
import { fireEvent } from '@testing-library/react'
fireEvent.click(screen.getByText(/Submit/))
π Security Best Practices in React
β οΈ Preventing XSS Attacks
Use dangerouslySetInnerHTML
cautiously and sanitize input with DOMPurify.
π‘οΈ CSRF Protection
Ensure secure authentication with CSRF tokens and strict CORS policies.
π The Future of React: Whatβs Next?
π React Concurrent Mode
Concurrent Mode enhances responsiveness by allowing React to work on multiple UI updates simultaneously.
π§ React Server Components
Improve performance by rendering components on the server before sending HTML to the client.
π Conclusion
React JS continues to evolve, providing developers with powerful tools to build scalable, high-performance applications. By mastering both basic and advanced concepts, optimizing performance, and following best practices, you can create React applications that are efficient, maintainable, and SEO-friendly.
π‘ Whatβs your favorite React feature? Share your thoughts in the comments! π
On this page
- π Introduction
- π― React JS Basics: Understanding the Fundamentals
- π Components and JSX
- π State and Props
- β‘ Virtual DOM
- π₯ Advanced React Concepts: Elevating Your Skills
- π React Hooks
- π Context API: Managing Global State
- π React Router: Client-Side Navigation
- π Optimizing React Performance
- ποΈ Memoization with useMemo
- π€ Lazy Loading with React.lazy
- π₯ Code Splitting for Faster Load Times
- π React Testing: Ensuring Code Quality
- β Unit Testing with Jest
- π Integration Testing with React Testing Library
- π Security Best Practices in React
- β οΈ Preventing XSS Attacks
- π‘οΈ CSRF Protection
- π The Future of React: Whatβs Next?
- π React Concurrent Mode
- π§ React Server Components
- π Conclusion
Discussion (0)
π Join the conversation!
Log in with GitHub or Google to share your thoughts and connect with the community.