Advanced MERN Stack Guide: Mastering Full-Stack Development
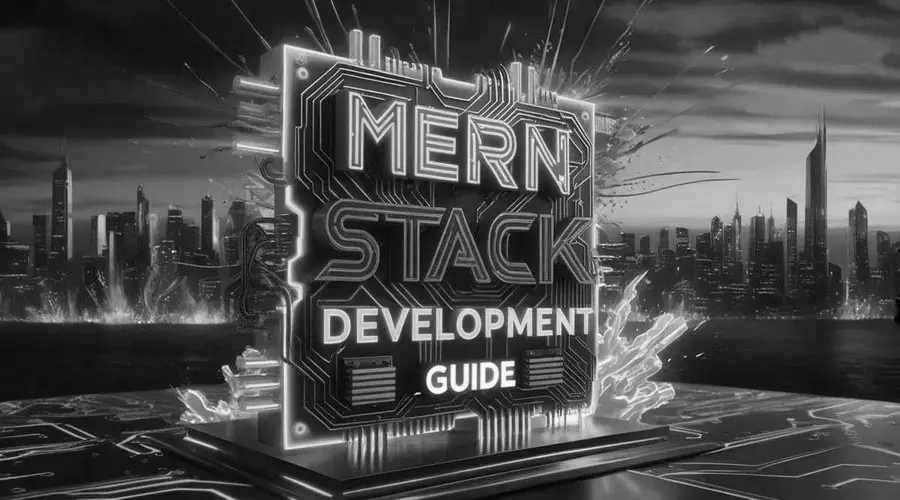
- Published on
- /4 mins read/––– views
The MERN stack (MongoDB, Express.js, React.js, Node.js) is a popular choice for building modern, scalable web applications. While many developers master the basics, transitioning to an advanced full-stack developer requires in-depth knowledge of performance optimization, security, scalability, and best practices.
This guide covers advanced techniques to help you build production-ready MERN applications with efficiency and scalability.
What is the MERN Stack?
The MERN stack is a full-stack JavaScript framework consisting of:
- MongoDB (NoSQL Database)
- Express.js (Backend Framework)
- React.js (Frontend Library)
- Node.js (Runtime Environment)
Together, these technologies enable developers to build fast, responsive, and scalable applications using JavaScript across the stack.
1. Advanced MongoDB Techniques
Optimizing Schema Design
- Embed vs. Reference: Embed related data for faster reads, use references for scalability.
- Leverage MongoDB Aggregation to process and transform data efficiently.
- Use Indexing (
Compound Indexes
,TTL Indexes
) to speed up query performance.
Scaling MongoDB
- Implement Sharding to distribute data across multiple nodes for horizontal scaling.
- Use MongoDB Atlas Performance Advisor to optimize query performance.
2. Express.js Best Practices
Efficient Middleware Architecture
- Use
helmet
,cors
, andcompression
for security and performance. - Implement custom middlewares for authentication, validation, and logging.
API Versioning & Documentation
- Version APIs (
/api/v1
,/api/v2
) to prevent breaking changes. - Document APIs using Swagger or Postman Collections.
Security Enhancements
- Sanitize inputs with
express-validator
. - Implement JWT-based authentication and refresh tokens.
- Use rate limiting (
express-rate-limit
) to prevent DDoS attacks.
3. Scaling React.js Applications
State Management
- Use Redux Toolkit, Recoil, or Zustand for global state management.
- Use React Query or Apollo Client for efficient server-state management.
Performance Optimizations
- Implement lazy loading and code splitting with React’s
React.lazy()
andSuspense
. - Use
React.memo()
and useCallback to prevent unnecessary re-renders. - Optimize images and assets using tools like ImageOptim and
react-virtualized
.
TypeScript Integration
- Migrate React components to TypeScript for better maintainability.
- Use strongly typed props and state to reduce runtime errors.
4. Advanced Node.js Backend Development
Optimizing Asynchronous Code
- Use async/await with proper error handling (
try/catch
orexpress-async-handler
). - Optimize performance with Node.js worker threads or clustering (
pm2
).
Logging & Monitoring
- Use Winston or Pino for structured logging.
- Set up real-time monitoring with New Relic, Datadog, or LogRocket.
Building Microservices
- Implement service-to-service communication using REST, GraphQL, or gRPC.
- Use Kafka.js or RabbitMQ for message queuing.
5. TypeScript in MERN Stack
- Convert both frontend and backend to TypeScript for type safety.
- Use Mongoose with TypeScript for strongly typed MongoDB models.
- Define API responses using Express Request Handlers with TypeScript.
6. Advanced Deployment Strategies
Containerization & Orchestration
- Use Docker for creating portable, containerized applications.
- Deploy to Kubernetes (K8s) for scaling and load balancing.
CI/CD Automation
- Use GitHub Actions, CircleCI, or Jenkins for automated deployments.
- Implement staging environments before pushing to production.
Cloud Hosting & Scaling
- Deploy with AWS Elastic Beanstalk, Vercel, or Google Cloud Run.
- Use Auto Scaling Groups and Load Balancers to manage high traffic.
7. Performance Optimization Techniques
Backend Optimizations
- Cache API responses using Redis.
- Use connection pooling in MongoDB (
mongoose.createConnection()
).
Frontend Optimizations
- Optimize CSS and JavaScript bundles with Webpack and Vite.
- Use tree shaking to remove unused code.
- Implement Lazy Loading and image optimization.
8. Essential Tools & Libraries
Development & Testing
- Postman/Insomnia for API testing.
- ESLint & Prettier for consistent coding style.
- Jest, Mocha, and Cypress for full-stack testing.
Authentication & Security
- OAuth with Auth0 or Firebase Authentication.
- Passport.js for session-based authentication.
- bcrypt for password hashing.
Final Thoughts
Mastering the MERN stack goes beyond just understanding its components—it requires knowledge of best practices, scalability techniques, and performance optimization.
By implementing the strategies in this guide, you’ll be well-equipped to build high-performance, scalable, and secure MERN stack applications. 🚀
Are you working on a MERN project? Let’s connect and discuss your challenges!
On this page
- What is the MERN Stack?
- 1. Advanced MongoDB Techniques
- Optimizing Schema Design
- Scaling MongoDB
- 2. Express.js Best Practices
- Efficient Middleware Architecture
- API Versioning & Documentation
- Security Enhancements
- 3. Scaling React.js Applications
- State Management
- Performance Optimizations
- TypeScript Integration
- 4. Advanced Node.js Backend Development
- Optimizing Asynchronous Code
- Logging & Monitoring
- Building Microservices
- 5. TypeScript in MERN Stack
- 6. Advanced Deployment Strategies
- Containerization & Orchestration
- CI/CD Automation
- Cloud Hosting & Scaling
- 7. Performance Optimization Techniques
- Backend Optimizations
- Frontend Optimizations
- 8. Essential Tools & Libraries
- Development & Testing
- Authentication & Security
- Final Thoughts
Discussion (0)
🚀 Join the conversation!
Log in with GitHub or Google to share your thoughts and connect with the community.